Detect Security Issues with Composer Audit and Gitlab CI
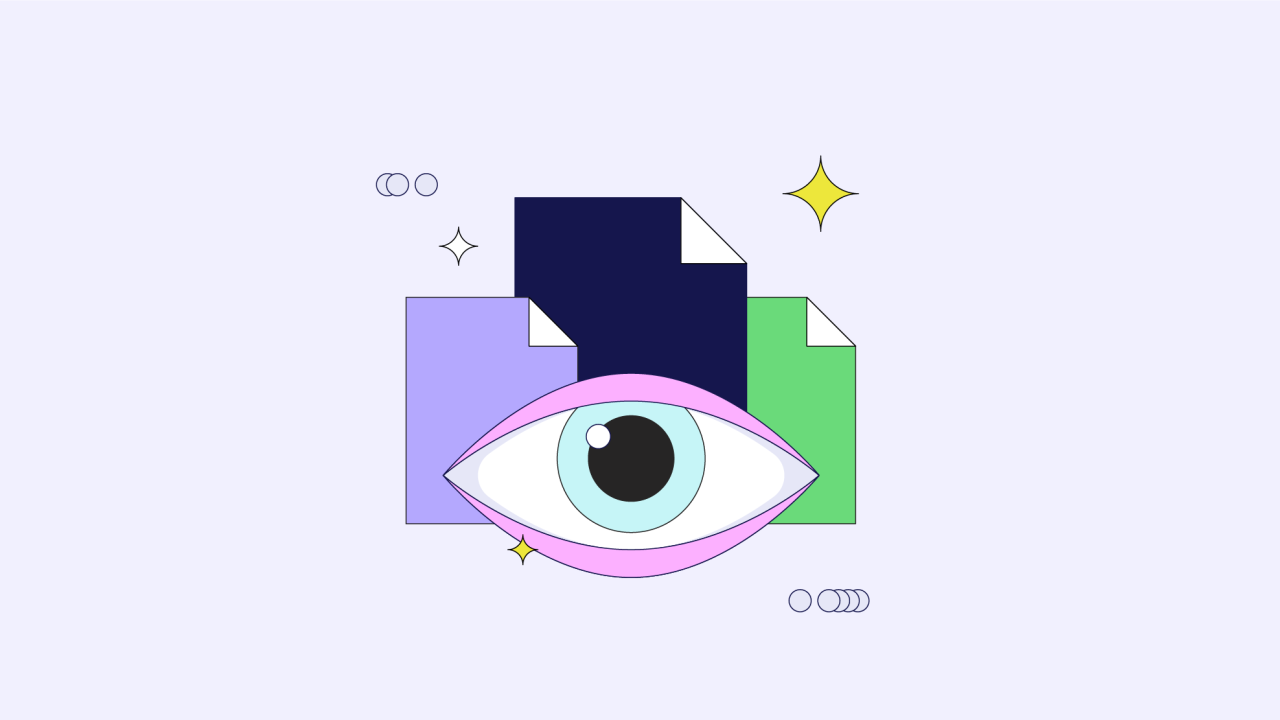
Application security is a key priority, especially in the face of growing cyber threats. To effectively monitor vulnerabilities in PHP dependencies, we have automated the auditing process by integrating Composer Audit with Mattermost and GitLab CI/CD. As a result, our team can respond instantly to threats in applications based on Drupal, Symfony, Grav, and WordPress—ensuring their stability and security.
Motivation
Our team's responsibilities include support, maintenance, vulnerability monitoring, and application security for our clients. Most of these are applications built on the Drupal platform, although we also occasionally work with other PHP-based applications, such as those built with Symfony, or simple websites based on systems like Grav or WordPress. To ensure the stability and security of these applications, regular updates are essential – especially when it comes to security-related updates.
Given the variety of platforms we deal with, we decided to create a simple yet universal method for identifying critical security update vulnerabilities. This allows us to respond quickly and provide the highest level of security for PHP-based applications under our maintenance.
Solution Philosophy
Before I get into the details, I want to outline the philosophical framework that guided our design process.
In his book “The Art of Unix Programming,” Eric S. Raymond succinctly summarized the key elements of Unix philosophy, emphasizing the benefits of small, composable programs.
The first description of this philosophy appeared in 1978, written by Doug McIlroy (article “Unix Time-Sharing System: Foreword”) in the “Bell System Technical Journal.” The Unix philosophy remains relevant and significant even 47 years later. In particular, the following principles resonate with us:
- Write programs that do one thing and do it well
- Write programs to work together
- Write programs to handle text streams, because that is a universal interface
Eric S. Raymond summarized this philosophy as “Keep it Simple, Stupid” (KISS). While preparing our solution, we strived to uphold the spirit of these principles.
Solution Components
- Composer Audit – We use the composer audit tool to generate a CVE report table. This allows us to identify known vulnerabilities in dependency packages managed in our composer.json.
- Mattermost – Our communication platform is Mattermost. It’s an open-source team communication platform and a great alternative to tools like Slack. We use the incoming webhooks mechanism to send vulnerability alerts.
- Bash Script – We wrote a simple script that integrates Mattermost and Composer Audit.
- Docker – We package the script along with its runtime environment in a Docker image. This allows us to easily move our tools between environments and ensure proper functionality.
- GitLab CI/CD – To scan periodically and during routine deployments, we use Ratioweb’s standard CI/CD solution – GitLab CI/CD. This allows us to continuously monitor the security status of our projects and respond quickly to any threats.
Implementation
Bash Script - entrypoint.sh
Below is the entrypoint.sh file, which acts as a wrapper for composer audit, enabling automatic vulnerability detection and team notifications via Mattermost.
#!/bin/sh
# Navigate to the project build directory (GitLab CI/CD variable)
cd $CI_PROJECT_DIR || exit 1
# Install dependencies defined in composer.json
composer install --ignore-platform-reqs
# Run the security audit. Ignore abandoned packages
auditResult=$(composer audit --abandoned=report --format=plain 2>&1)
# The script returns the number of packages listed in the report
auditStatus=$?
# Check if COMPOSER_SECURITY_MATTERMOST_WEBHOOK is set and we have at least one vulnerability
if [ -n "${COMPOSER_SECURITY_MATTERMOST_WEBHOOK}" ] && [ $auditStatus -ge 1 ]; then
# Send the audit result to the Mattermost webhook
jsonPayload=$(printf '{"text": "%s", "priority": { "priority": "important", "requested_ack": true }}' "### Security Report: $CI_PROJECT_PATH\n$auditResult")
echo "$jsonPayload" | http --verify=no POST "${COMPOSER_SECURITY_MATTERMOST_WEBHOOK}"
else
echo "$auditResult"
fi
exit $auditStatus
Let’s summarize the steps of entrypoint.sh
- Navigates to the project directory
cd $CI_PROJECT_DIR || exit 1
– exits if the directory doesn’t exist.
- Installs dependencies from composer.json
composer install --ignore-platform-reqs
– ignores platform requirements (e.g., php extensions). We don’t execute the code, just perform static analysis.
- Performs the security audit
auditResult=$(composer audit --abandoned=report --format=plain 2>&1)
checks for vulnerabilities. Information about abandoned packages will be shown but won’t affect audit results.
- Analyzes the audit result
- If vulnerabilities are found and a Mattermost webhook is set → sends an alert and exits with error.
- If vulnerabilities are found but webhook is not set → exits with error and prints the report in console
- No vulnerabilities – prints the result to the console.
Dockerfile
To run our script in different environments, we package it in a Docker container. Here is the Dockerfile
:
ARG PHP_VER
FROM php:$PHP_VER-alpine
RUN apk update && apk add httpie git unzip patch
# Download and install Composer
RUN curl -sS https://getcomposer.org/installer | php -- --install-dir=/usr/local/bin --filename=composer
RUN chmod +x /usr/local/bin/composer
COPY entrypoint.sh /usr/local/bin/entrypoint.sh
ENV COMPOSER_SECURITY_MATTERMOST_WEBHOOK ""
ENTRYPOINT ["/usr/local/bin/entrypoint.sh"]
How does this Dockerfile work?
- Based on the official PHP Docker image from hub.docker.com
FROM php:$PHP_VER-alpine
– uses the lightweight Alpine image for specific PHP versions.
- Installs additional tools
apk add httpie git unzip patch
– adds required system packages.
- Downloads and installs the latest Composer version
curl -sS https://getcomposer.org/installer | php
– downloads and installs Composer.chmod +x /usr/local/bin/composer
– grants execution permission.
- Adds bash audit script
COPY entrypoint.sh /usr/local/bin/entrypoint.sh
– copies the script into the container.
- Sets environment variable placeholder
ENV COMPOSER_SECURITY_MATTERMOST_WEBHOOK ""
– placeholder for Mattermost webhook.
- Defines the entry point
ENTRYPOINT ["/usr/local/bin/entrypoint.sh"]
– script runs at container startup.
How do we build our Docker image?
We build this image for each supported PHP version (e.g., 8.1, 8.2, 8.3), using the following script:
docker build -t "$REPO":"$PHP_VER" --cache-from "$REPO":"$PHP_VER" --build-arg PHP_VER="$PHP_VER" .
- REPO is the Docker image registry URL (e.g., private Docker repo on our private GitLab instance)
- PHP_VER is one of the supported PHP versions (e.g., 8.1)
GitLab CI/CD Integration
To ensure automatic vulnerability detection in PHP dependencies, we integrated with GitLab CI/CD. We use two types of jobs:
- test:composer-audit – checks for vulnerabilities in interactive pipelines (e.g., within merge requests).
- security:composer – runs as a scheduled job and scans for new vulnerabilities daily.
GitLab CI/CD Configuration
Below is a fragment of the .gitlab-ci.yml
file with our CI/CD pipeline configuration.
security:composer:
stage: tasks
image: {private-registry-url}/toolkit/composersecurity:$PHP_VERSION
needs: []
script:
entrypoint.sh
rules:
- if: $CI_PIPELINE_SOURCE == "schedule"
exists:
- '**/composer.json'
-
when: manual
exists:
- '**/composer.json'
test:composer-audit:
extends: security:composer
stage: test
variables:
COMPOSER_SECURITY_MATTERMOST_WEBHOOK: ""
allow_failure: true
rules:
- if: '$CI_PIPELINE_SOURCE != "merge_request_event"'
when: never
- exists:
- '**/composer.json'
How the jobs in .gitlab-ci.yml work:
- test:composer-audit
- Runs only in merge request context
- Allows developers to check for vulnerabilities before deployment
- Mattermost webhook is disabled (COMPOSER_SECURITY_MATTERMOST_WEBHOOK="") to avoid unnecessary alerts
- If vulnerabilities are found, the script fails, but doesn't block deployment (
allow_failure: true
)
- security:composer
- Runs once daily as a GitLab scheduled job (https://docs.gitlab.co.jp/ee/ci/pipelines/schedules.html)
- If a new vulnerability is found, it sends a notification to the maintenance team.
Using this mechanism, we run the scanner once per day. This way, whenever a new vulnerability is discovered, the tool will inform the maintenance team about the needed update to one of the managed projects.
Summary
Why this solution?
Automated vulnerability verification, GitLab CI/CD integration, and real-time notifications via Mattermost create a comprehensive security protection mechanism for PHP applications. This allows our team to respond quickly to threats and minimize the risk of attacks. Docker ensures flexibility and ease of deployment across various environments, further boosting the solution's efficiency.
What have we achieved?
- Automated security auditing – Composer Audit allows us to detect vulnerabilities in PHP dependencies.
- GitLab CI/CD integration – Regular daily scans and merge request auditing ensure continuous security control.
- Mattermost notifications – Automated alerts allow the team to respond quickly to detected threats.
- Portability and easy setup – Thanks to Docker, our tool works in different environments without additional configuration.
Benefits for the maintenance team
- Proactive security approach – Monitoring for new vulnerabilities allows for a rapid response and minimizes attack risks.
- Full integration into the application lifecycle – The tool supports both ongoing maintenance and application development without interfering with deployment processes.
- Clear communication – Automated reports reduce the risk of missing vulnerabilities and help plan updates efficiently.
Benefits for the client
- Secure applications – Regular audits eliminate known vulnerabilities, providing greater protection against attacks.
- Minimized downtime risk – Automatic detection and resolution reduce the likelihood of system failures.
- Compliance with best practices – Auditing supports adherence to security requirements, which may be crucial for regulatory and industry standards.
- Greater software stability – Ongoing updates help maintain high quality and reliability of applications.
With this architecture, we provide the highest level of security for applications built with Drupal, Symfony, Grav, and WordPress, eliminating the need for manual vulnerability analysis.
Automated security verification processes, GitLab CI/CD integration, and real-time Mattermost notifications form a comprehensive solution that enables rapid response to threats and minimizes attack risks. Thanks to Docker, our tool is flexible and easy to deploy across multiple environments, making it even more effective.
The application maintenance team gains a tool that not only enhances security but also streamlines the entire process of update management and threat detection, enabling fast reactions to emerging issues.
ambitious projects and people