Frontend toolbox: Gulp
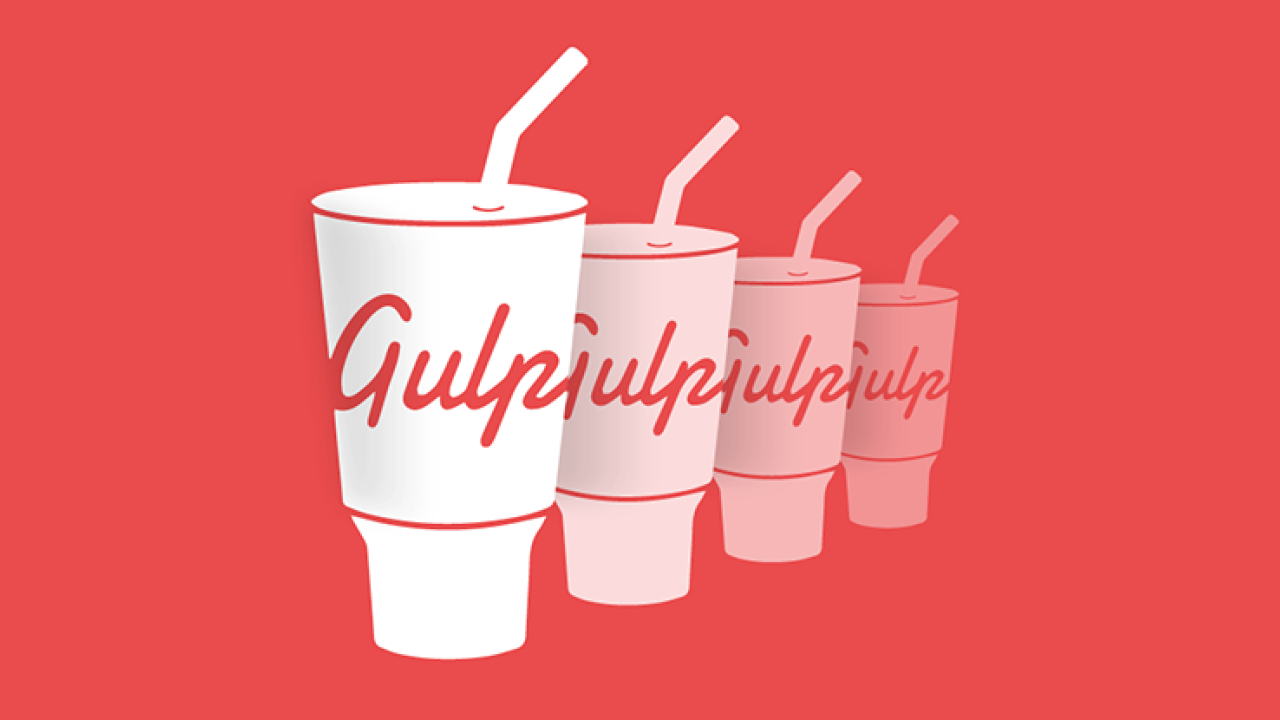
Repetitive tasks such as SCSS compilation, code minification or automatic browser refreshes can consume valuable time. Gulp solves this problem by allowing you to fully automate these processes. In this article, you will find configuration examples and practical applications of Gulp to streamline your workflow.
What is Gulp?
In simple words, Gulp is a task runner which allow us to automate repetitive actions so we can concentrate on coding.
The best and fastest way to learn about its capabilities is to check all available gulp plugins.
From my experience, the first thing developer finds useful is the watch function which observes our scss and javascript files for changes and then runs defined tasks on it.
Example tasks can be:
- Convert .scss or .less files into .css ones,
- Prefixing css rules (e.g. display: flex) so we can forget about add prefixes manually,
- Sourcemap generator, which allows us to inspect source .scss files in the browser just like we can inspect .css ones,
- Minify css and javascript files,
- Automatically generate image sprites,
- Concatenate many javascript files into one final script,
- Auto refresh browser.
There are many different tasks to do, but we can just use one $ gulp watch
command and everything mentioned above will do in the background during coding.
How to use Gulp?
First of all, you have to install node.js and npm. To achieve this you can follow the official guide, or use nvm (which is the acronym of Node Version Manager). I prefer the latter because I can easily switch between node versions, depends on specific project’s requirements.
Now install Gulp with command: $ sudo npm install -g gulp
The -g flag will install Gulp globally which means you can use $ gulp command in your console.
Now, you can add Gulp into your project by two ways (I assume you already use npm):
1. Add Gulp by running $ npm install --save-dev gulp
command. It will create an empty gulpfile.js. Now you can install any gulp plugin using npm.
2. Take any existing gulpfile.js and copy it into the project. You also have to look at required plugins and install them.
Example of use
Below you can see a practical example of gulpfile.js. The defined tasks are responsible for converting .scss into .css files, merge all .scss files into one .css, and to add browser-specific prefixes for some css rules.
To run this configuration you have to `npm install --save-dev` (or add to package.json) following gulp plugins:
- gulp-sass
- gulp-autoprefixer
- gulp-concat
- merge-stream
- gulp-shell
- gulp-sourcemaps
var gulp = require("gulp");
var sass = require("gulp-sass");
// Additional configuration and import node libraries
var sassOptions = {
errLogToConsole: true,
outputStyle: 'expanded',
};
// Allow to inspect scss files in browser's css inspector.
var sourcemaps = require('gulp-sourcemaps');
var shell = require('gulp-shell');
// Automatically create browser-related prefixes in CSS rules.
var autoprefixer = require('gulp-autoprefixer');
var concat = require('gulp-concat');
var merge = require('merge-stream');
//Supported browsers
var autoprefixerOptions = {
browsers: ['last 2 versions', '> 5%', 'Firefox ESR']
};
// Convert all .scss files into single .css
gulp.task('sass', function() {
return gulp.src('./scss/**/*.scss')
.pipe(sourcemaps.init())
.pipe(sass(sassOptions).on('error', sass.logError))
.pipe(autoprefixer(autoprefixerOptions))
.pipe(concat('style.css'))
.pipe(sourcemaps.write('../maps'))
.pipe(gulp.dest('./css'));
});
// Process JS files and return the stream.
gulp.task('js', function() {
return gulp.src('js/*js')
.pipe(gulp.dest('js'));
});
// Default task to be run with single `gulp` command.
// By default we want to watch for changes in our .scss and .js files and then run defined tasks.
gulp.task('default', ['sass', 'js'], function() {
gulp.watch("scss/**/*.scss", ['sass']);
gulp.watch("js/*.js", ['js']);
});
Summary
As you can see Gulp is a very simple tool which can make an impact on your productivity and quality of your code. The second important thing is that Gulp kind of tool from category “configure once and forget” - you only need to instruct your team to use one command `gulp watch` and they can use every tool I implemented by gulp plugin.
ambitious projects and people